Unit Test Angular FullCalendar with Jest
Here's how to fix a common error that happens when you use Jest with Angular FullCalendar.
•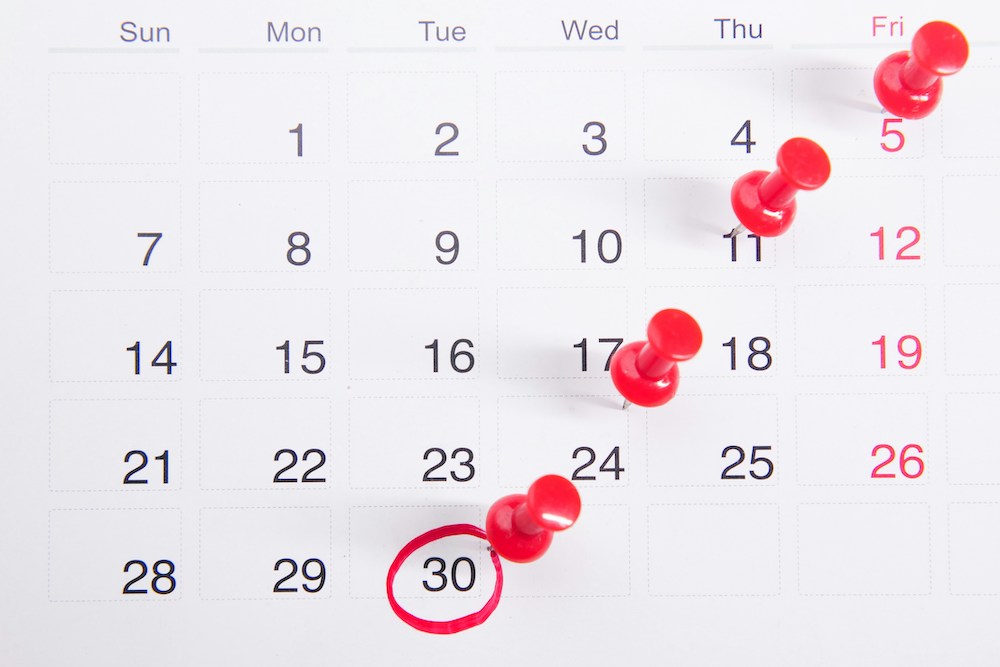
When you use FullCalendar in an Angular Project, you might see an error that looks like this.
Jest encountered an unexpected token
Jest failed to parse a file. This happens e.g. when your code or its dependencies use non-standard JavaScript syntax, or when Jest is not configured to support such syntax.
Out of the box Jest supports Babel, which will be used to transform your files into valid JS based on your Babel configuration.
By default "node_modules" folder is ignored by transformers.
SyntaxError: Unexpected token 'export'
Here's how to fix that error.
1. Open Your Jest Config File - jest.config.ts
and add the following transformIgnorePatterns
import type { Config } from 'jest'
const config: Config = {
preset: 'jest-preset-angular',
setupFilesAfterEnv: ['<rootDir>/setup-jest.ts'],
transformIgnorePatterns: ['node_modules/(?!(.*.mjs$|@fullcalendar|preact))']
}
export default config
Now if you run your unit tests you might see an error that starts with
Error during cleanup of component
2. Open The Unit Test
and add fixture.detectChanges()
it('should create the app', () => {
const fixture = TestBed.createComponent(AppComponent);
const app = fixture.componentInstance;
fixture.detectChanges();
expect(app).toBeTruthy();
});
Now you won't see any errors, and you can continue to unit test your Angular FullCalendar project.